How automation saved my relationship (🪤)
Oh, we're not done with Script Kit yet. There's still stuff we can automate!
Context
I pride myself in being a caring and attentive partner. I set little reminders to check in with my girl to see how she’s doing; we have a monthly check-in that we engage in where we report on the status of the relationship and how we’re feeling about things (🔥 so hot right); and I do my utmost to try and deescalate any situations if I’m ever feeling like it’s getting a little too heated. There’s good kinds of 🔥 and bad kinds of 🔥, if ye know what I mean.
However, I am far from perfect. One of the things I struggle with the most — like a lot of you fine folks reading this article — is pulling myself away from the computer when I’ve entered the flow state. If you don’t know about the flow state, this blog post does a good job of going over it and why it rocks! The TLDR is that you’re in the zone and you don’t want to leave the zone because the zone is where sh*t gets done. Now, if you add in the inability to rip myself away from the screen when I’m in flow and an emergency 🚨 in production, I’ll almost always forget to message my girl that I’ll be working late. In fact, I probably will not be responding to any messages she sends my way so her “just checking in on how your day is going” messages go totally ignored.
Again, I just said, I pride myself in being a caring and attentive partner. Whilst flow state is a great thing, it’s not the most important thing in my life and human relationships matter way more to me than any issue in production. Ignoring your partner is not a good thing (obviously) and in fact — and don’t quote me on this but I swear I heard it from someone — being ignored and feeling ignored is as bad for as you as smoking a cigarette! Again, don’t quote me on this and when I Googled it, I couldn’t find squat, but we’re gonna run with it because even if it’s not true, it really really sucks being ignored.
Alright, so leaving my girl hanging wasn’t an option anymore. I needed a solution that could pull me out of the flow state gently but effectively and ensure I stayed the caring and attentive partner I aspire to be.
As a developer, the obvious choice was to code my way out of this problem. After all, if tech can solve production emergencies, why not relationship ones too? Enter Script Kit and a little help from Twilio. Here’s how I hacked my way out of the dog house and into “legendary” boyfriend status.
A Demo
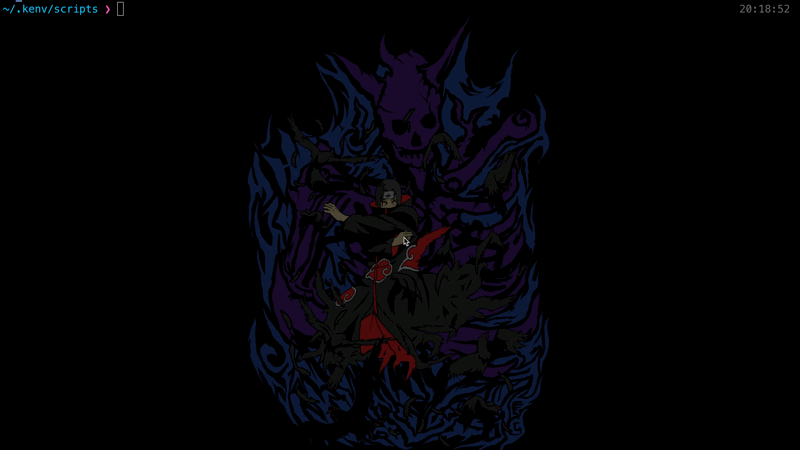
The Code (Since I Know That’s What You Want)
// Name: Whatsapp Babe A Late Message
// Description: Send your babe a whatsapp templated mesage to let em know you're running late!
// Author: Taran "tearing it up" Bains
// GitHub: @tearingitup786
// Schedule: 0 14 * * *
import '@johnlindquist/kit' // ScriptKit imports
// Import Twilio SDK
const twilio = await npm('twilio')
const phoneNumbers = await env(
'TWILIO_PHONE_NUMBERS',
'comma separated list of numbers: 131-131-1313,787-787-7878',
)
try {
const numbersToText = phoneNumbers.split(',')
// Your Twilio Account SID
const accountSid = await env('TWILIO_ACCOUNT_SID')
// Your Twilio Auth Token
const authToken = await env('TWILIO_AUTH_TOKEN')
// Your Twilio phone number
/**
* You'll have to set up a whatsapp/facebook business account
* Twilio makes it pretty easy to do this so don't fret, it's pretty straightforward.
*
* @docs https://www.twilio.com/docs/whatsapp/getting-started
*/
const fromNumber = await env('TWILIO_PHONE_NUMBER')
/**
* We opt for a message template so that the numbers we are messaging
* don't need to have this particular number saved as a contact
* We're able to start conversations with whatsapp users with message template
* doing it via adhoc messaging doesn't really work!
* @docs https://www.twilio.com/docs/whatsapp/tutorial/send-whatsapp-notification-messages-templates
*/
const contentSid = await env('TWILIO_CONTENT_SID')
const workingLate = await arg('Are you working late', ['yes', 'no'])
if (workingLate === 'no') {
console.log("Okay, won't text babe!")
process.exit()
}
// Initialize Twilio client
const client = twilio(accountSid, authToken)
// Function to send a message
const phoneNumber = await arg(
"Enter the recipient's phone number (e.g., +1234567890):",
numbersToText,
)
const messageBody = await arg(
"Enter when you'll be free... He'll be free around <your message>",
)
console.log('Last time given', messageBody)
client.messages
.create({
// body: messageBedy,
contentVariables: JSON.stringify({1: `${messageBody}`}),
from: `whatsapp:${fromNumber}`,
contentSid,
to: `whatsapp:+1${phoneNumber}`,
})
.then(message => {
notify(`Message sent to ${phoneNumber}! Message SID: ${message.sid}`)
console.log(`Message SID: ${message.sid}`)
})
.catch(error => {
notify(`Error sending message: ${error.message}`)
console.error('Error:', error)
})
} catch (err) {
console.error('There was an error running the twilio script')
console.error('Error', err)
}
The Setup
So before we jump into Twilio, I think you should go read my blog post about Script Kit! I’ll wait. Okay, you probably didn’t read it so I’ll give you the TLDR. The TLDR; is that it’s a scripting platform intended to help developers automate tedious parts of their workflow. BTW, if you’re reading this babe, nothing about you is tedious 😰. I swear, if this blog post gets brought up in a future fight, even as a joke, this is going to be me:
Why Twilio?
Now, I needed a reliable way to send a perfectly-timed text to my girlfriend. Sure, I could’ve stuck with reminders, but where’s the fun in that? Twilio came to the rescue with its robust API, making it effortless to send SMS messages programmatically. It fit seamlessly into my Script Kit workflow, bridging the gap between code and communication.
So this isn’t a blog about Twilio but if
you haven’t heard about it before, it’s a cloud communications platform that
allows you to send not only emails and SMS messages programmatically, but also,
and this is relevant to my blog post, WhatsApp
messages 👏! I went with Twilio
over another provider because I’ve got experience with their platform and they
had the coveted WhatsApp
integration that I needed.
The setup for Twilio is as following:
- Sign up for Twilio.
- Get a phone number (~$1.15 USD).
- Add funds for messaging (~$0.025 per WhatsApp message).
The things you’ll need to run the script from Twilio can be found in the dashboard. They are the following:
- Account SID
- Auth Token
- Phone number
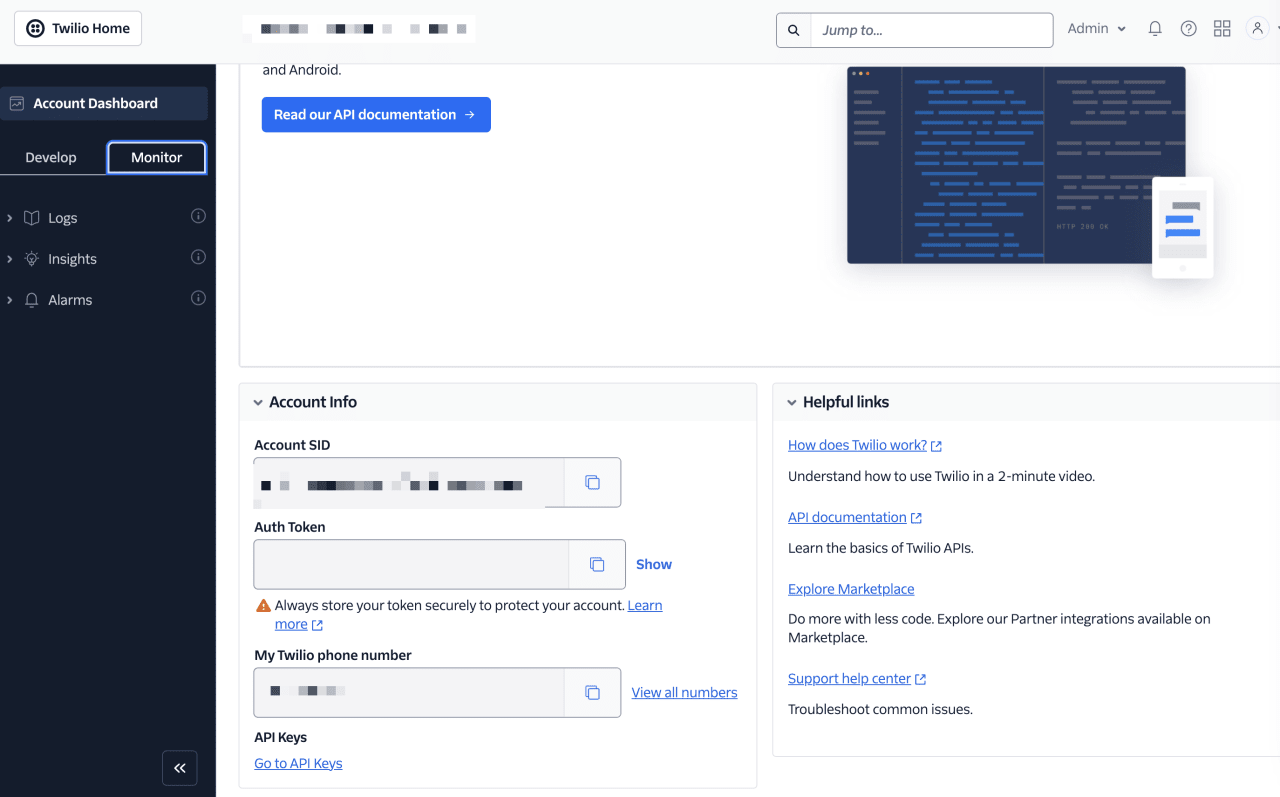
If all you’re wanting to do is send SMS messages, you’re all set. You’ll just
need to tweak the script and remove any references to whatsapp
in the from
and to
fields in the create
method (line 70
and 72
respectively)! If
you’re running the full gambit, you’ll also have to create a WhatsApp Sender
inside of Twilio.
From what I recall, the only difficult part, and I use the word difficult
liberally here since nothing is really all that hard when it comes to this
automation, is creating your Facebook business profile. I called my business
Taranveer Bains Business — that’s it, just my name, followed by business 😆.
Assuming everything goes well with creating Facebook business and your
WhatsApp Sender
, the next thing you’ll most likely want to do, is create a
whatsapp template. The primary benefit I’ve seen with using the template versus
a traditional message is that by using a template, your recipient will not have
to save your phone number in order to receive messages. For example, when I
tried to message my own phone number with my automation, I wasn’t able to
actually receive the messages until I had saved the number in my contacts.
This wasn’t great because I didn’t want to create unnecessary work for my girl.
There were a few other issues I ran into but I’ll cover them later. Check out
the video below to see how easy it is to create a message template!
The Issues I Ran into
Okay so this one is on me for not knowing the limitations of the platform, and this makes perfect logical sense: Twilio stop sending my messages to my gf. This happened, not because I voided their terms of service or anything like that, but because, my gf didn’t respond to any of my messages. This most likely flagged my messages as “spammy” because I was broadcasting one way and not getting any engagement.
The fix to my messages not being delivered was to ask my gf to respond every time she got a message. That isn’t the ideal fix but it’ll do for now.
Next Steps
Like I said, it’s kinda lame that my gf has to respond to my Twilio number to stop the number from being flagged. It’s also lame that she sends a message to the number and that’s the end of it — it just goes straight into the void ⚫. It would be super cool if I had a way of receiving said messages so that I know it was delivered and that she’s actually cool with me working late. From what I gather, it actually isn’t all that hard to set up a service to do this. From my cursory research, it seems like I need to associate a webhook with my number in the Twilio dashboard and have a server sitting somewhere listening for messages and then performing some action when a message comes through. Now… if only I had a server running somewhere where I could do this 🤔. Oh wait! I have my remix site (the one you’re reading this post on), so maybe I can just create a resource endpoint here and be about my business.
I haven’t done it yet, but that’s the plan. I’ll come and update this blog post once I’ve done that part!
Closing Thoughts
Scripting isn’t just about automating tasks or writing clever code—it’s about reclaiming time and mental bandwidth to focus on what truly matters. Whether it’s sending messages to your girl one or streamlining repetitive workflows (like checking if your enterprise company’s website version has updated), automation is a tool that empowers us to be more present, thoughtful, and efficient.
Shout out to my girl for being the love of my life and inspiring this automation ❤️.
Love is not only about what we can get, but what we can give.
-A. M. S.